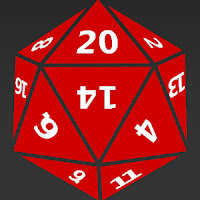
I like to compare frameworks with multi sided cubes...
Each side of the cube exposes a key aspect of the framework morphology.
The collection of all aspects is often called a Meta Model.
What are the various aspects that come to mind when thinking about User Interface Framework Design?
In this post, you will see the ones that I find important...
You can use it as a check list or reflection board when you plan on writing your own framework.
Feel free to comment on this post if you have remarks or like to share some thoughts of your own.
I. Data Model
Data Representation
- What Objects does your framework use to represent Data (PODOs, Value Objects, ...)?
Meta Data
- What is the Meta Data model of your Data Object (field definition, field data types, field default values, ...)?
Data Access
- How can your framework read / insert / update / delete data and invoke remote view actions (database queries, web services, ...)?
Data Validation
- Does your framework support Data Validation (definition and enforcement of Validation rules: not null, min / max length, email address, telephone number, ...)?
Data Transformation
- Does your framework support Data Transformation (definition and enforcement of Transformation rules: lowercase / uppercase / numeric only, ...)?
Calculated Fields
- Does your framework support Calculated Fields?
Cursors & Pagination
- How does your framework implement navigation and pagination through small and big data sets?
II. User Interface
Data Binding
- How does your framework bind Data Objects to UI Components (does it have support for distributing change to multiple observers)?
UI Components
- How does your framework present Data Object values for editing / visualisation (text fields, combo boxes, checkboxes, date pickers, tables, trees, charts, ...)?
UI Component Configuration
- Does your framework support configuring UI Components (enabled / disabled / visible / invisible, value format, colours, font...)?
UI Containers
- Does your framework support creation of UI Component compositions (panels, tabbed pane, split pane, ...)?
UI Screens
- Does your framework support creation of functional / logical pages of UI Components and Containers?
III. Behaviour
Social Behaviour
- How does your framework allow UI Components to interact with each other (auto change values / colours / fonts, enabling / disabling / hiding / showing of a UI Component in reaction to a change of a neighbour UI Component)?
Scenarios
- How does your framework enable UI Component Containers, Screens and Data Access to interact with each other?
View Actions
- Does your framework allow the User to invoke certain view actions on the server (buttons, popup menus, ...)?
Exception Handling
- How does your framework handle Exceptions?
IV. Applications
Standard Functionality
- What standard functionality does your framework support (refresh, insert, update, delete, save, print data, import data, export data, undo, redo, cut, copy, paste, delete, ...)?
Application Security
- How does your framework implement authentication, authorisation & auditing?
Navigation
- How does your framework support navigation between Screens (menu of screens, search screen, ...)?
Global State
- Does your framework support Global Application State (application context)?
External Applications
- Does your framework (use or) support interaction with other external applications?
V. User Preferences
Look & Feel
- Does your framework support multiple (configurable) look and feels?
Customisations
- Does your application support screen customisation per user (dynamically assemble screen UI Components, define a list of favourite screens, show User recent visits, remember sorting and ordering of table columns, user specific default values for search criteria, ...)?
Internationalisation
- Does your framework have multi language support?
VI. Development
Application Configuration
- How to implement or configure applications in your framework (define screens, data model, data access, behaviour, ...)?
Custom Implementations
- Is there support for overriding certain parts of the framework to implement custom behaviour (modular architecture, anchor points, overriding functionality, ...)?
Software Factory
- How to do Rapid Application Development?
Mind Map
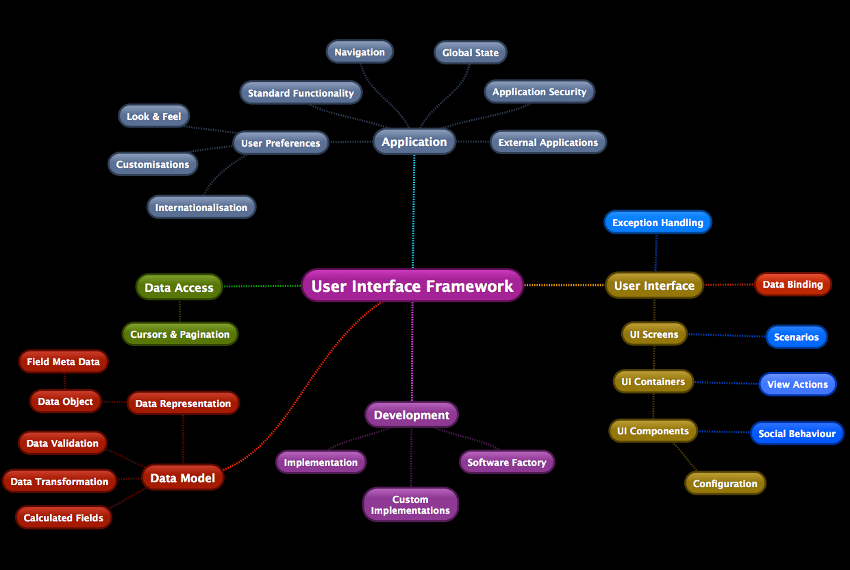
Links
https://en.wikipedia.org/wiki/Metamodeling
https://en.wikipedia.org/wiki/Model-driven_software_development
http://www.w3.org/TR/mbui-intro/